30 Day Vanilla JS Coding Challenge Study - 7주차
- How JavaScript’s Array Reduce Works
- Unreal Webcam Fun with getUserMedia() and HTML5 Canvas
- Speech Recognition
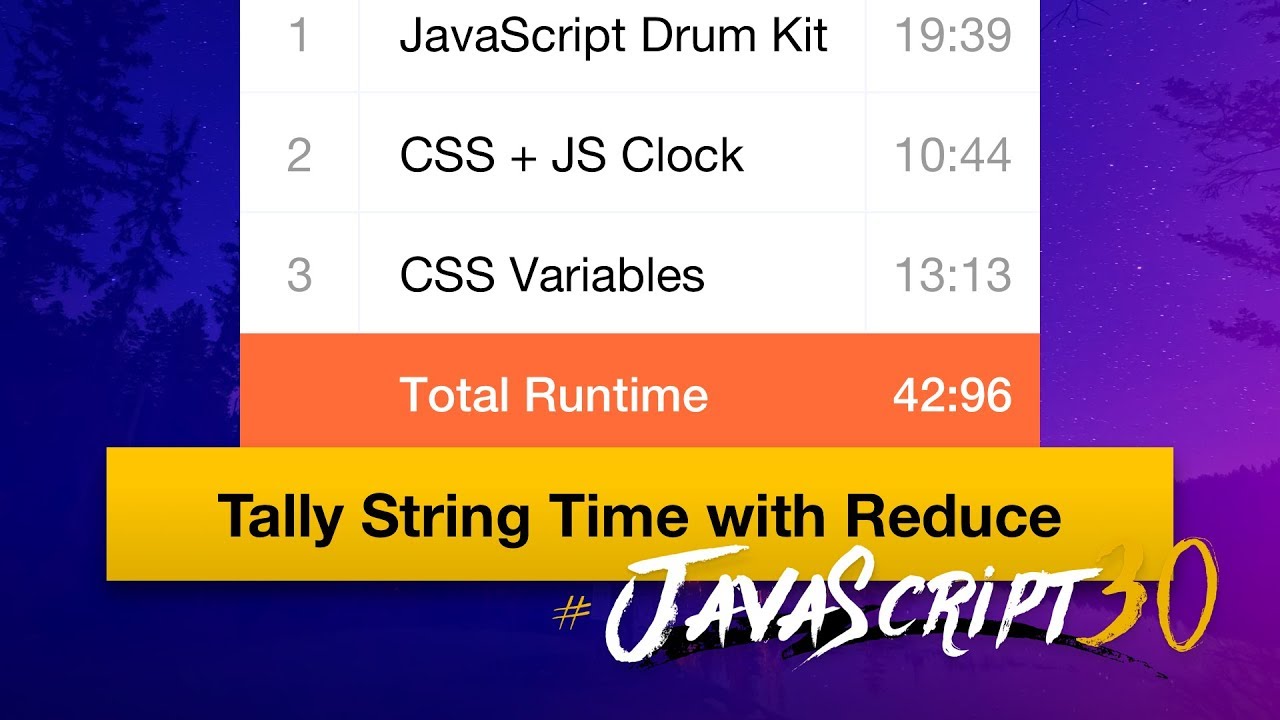
18. How JavaScript’s Array Reduce Works
영상: https://youtu.be/SadWPo2KZWg
reduce
메서드의 활용 방법
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| const timeNodes = Array.from(document.querySelectorAll('[data-time]'));
const seconds = timeNodes .map(node => node.dataset.time) .map(timeCode => { const [mins, secs] = timeCode.split(':').map(parseFloat); return (mins * 60) + secs; }) .reduce((total, vidSeconds) => total + vidSeconds);
let secondsLeft = seconds; const hours = Math.floor(secondsLeft / 3600); secondsLeft = secondsLeft % 3600;
const mins = Math.floor(secondsLeft / 60); secondsLeft = secondsLeft % 60;
console.log(hours, mins, secondsLeft);
|
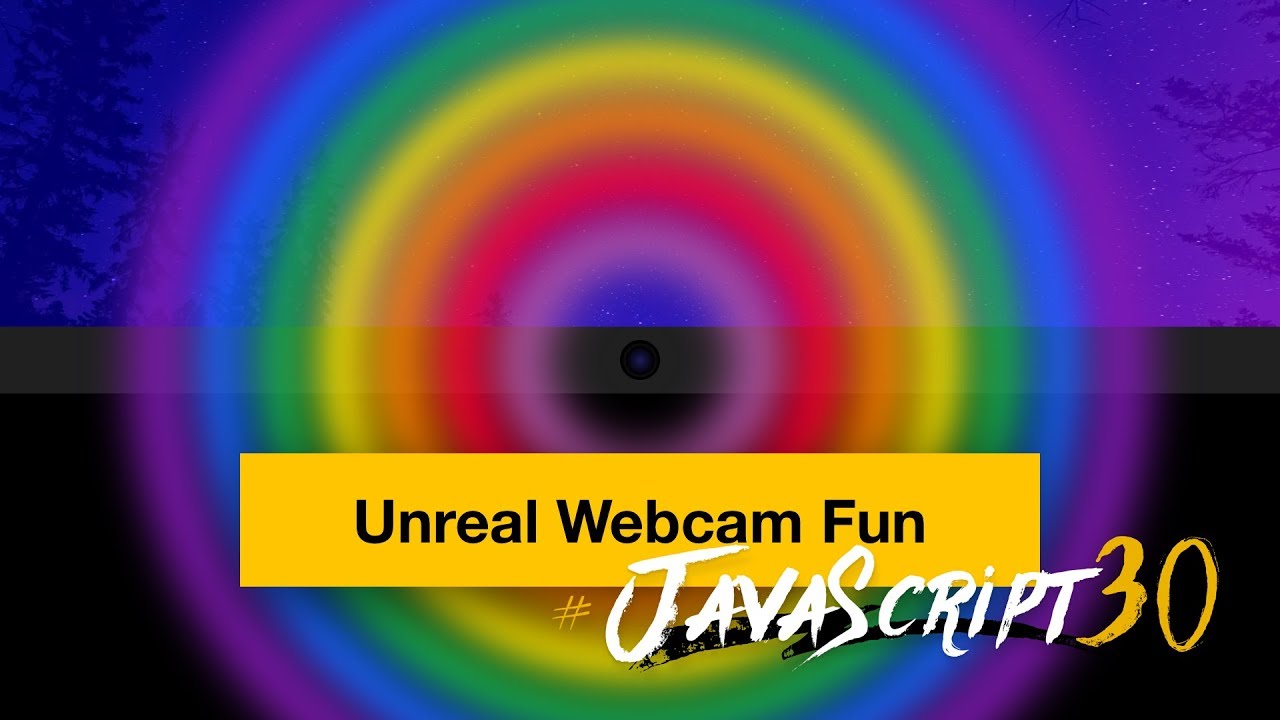
영상: https://youtu.be/ElWFcBlVk-o
getUserMedia()
와 HTML5 캔버스를 활용
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| function getVideo() { navigator.mediaDevices.getUserMedia({ video: true, audio: false }) .then(localMediaStream => { video.srcObject = localMediaStream; video.play(); }) .catch(err => { console.error(`OH NO!!!`, err); }); }
video.addEventListener('canplay', paintToCanvas);
|
이외에 로직은 RGB 값 및 캔버스 조작과 takePhoto()
함수를 통한 로직
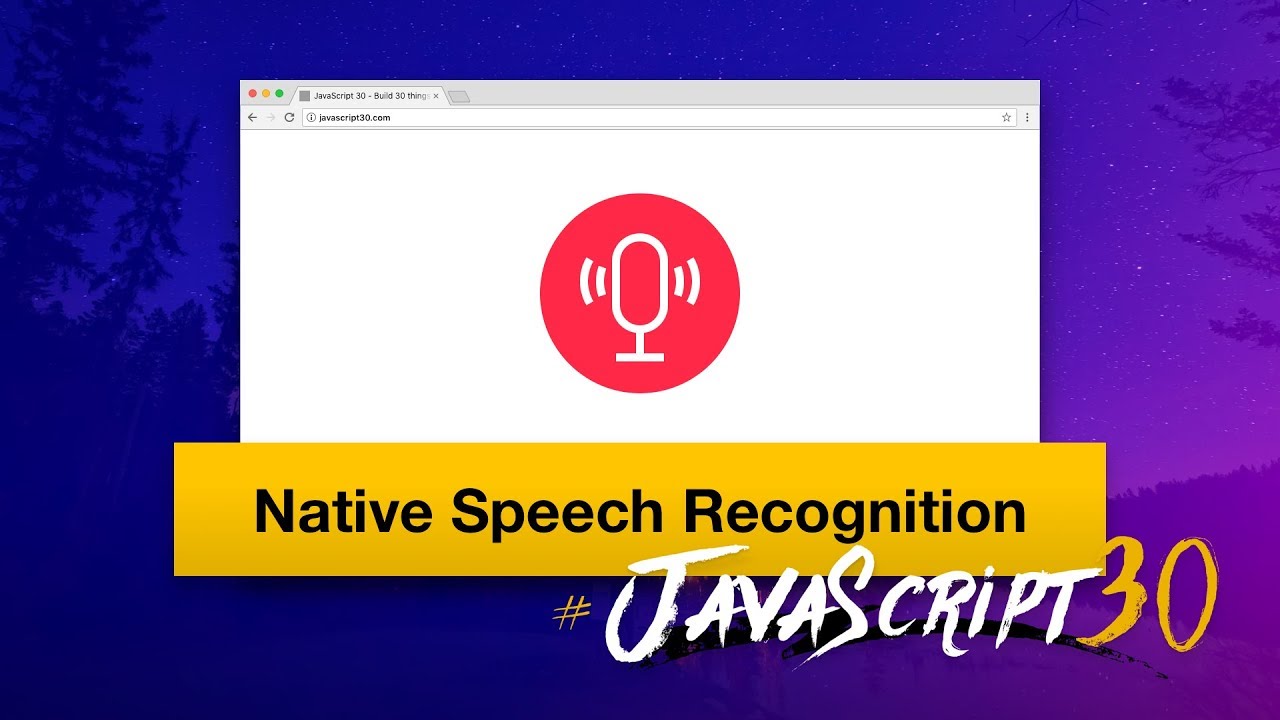
20. Speech Recognition
영상: https://youtu.be/0mJC0A72Fnw
Web Speech API를 활용한 음성인식
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| window.SpeechRecognition = window.SpeechRecognition || window.webkitSpeechRecognition;
const recognition = new SpeechRecognition();
recognition.interimResults = true; recognition.lang = 'en-US';
let p = document.createElement('p'); const words = document.querySelector('.words'); words.appendChild(p);
recognition.addEventListener('result', e => { const transcript = Array.from(e.results) .map(result => result[0]) .map(result => result.transcript) .join('');
const poopScript = transcript.replace(/poop|poo|shit|dump/gi, '💩'); p.textContent = poopScript;
if (e.results[0].isFinal) { p = document.createElement('p'); words.appendChild(p); } });
recognition.addEventListener('end', recognition.start);
recognition.start();
|